MetaMask Web3 Front-End Integration: A Beginner’s Guide
- aqib khan
- July 9, 2024
- No Comments
MetaMask Web3 Front-End Integration: A Beginner's Guide
In this article, we will walk you through creating a simple web project that integrates MetaMask, Web3.js, and Bootstrap to provide a stylish user interface for connecting a wallet and sending Ether. This guide is suitable for beginners and covers everything from setting up the HTML structure to adding JavaScript functionality. By the end of this guide, you will also gain an understanding of Web3.js and its role in interacting with the Ethereum blockchain.
Prerequisites
Before we start, ensure you have the following:
1. A basic understanding of HTML, CSS, and JavaScript.
2. MetaMask installed in your browser. If not, you can download it from [MetaMask’s website](https://metamask.io/).
Project Overview
Our project will:
1. Include a Bootstrap-styled pricing card.
2. Allow users to connect their MetaMask wallet.
3. Send Ether to a specified address.
4. Display messages based on the success or failure of operations.
Let’s break down the process step by step.
Step 1: Setting Up the HTML Structure
We’ll start by creating the HTML structure for our project. This includes a Bootstrap card for displaying the pricing plan, buttons for connecting the wallet and sending Ether, and a form for project submission.
Create an `index.html` file and add the following code:
html
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Connect Wallet and Send Ether</title>
<link href=”https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css” rel=”stylesheet”>
<script src=”https://code.jquery.com/jquery-3.6.0.min.js”></script>
<script src=”https://cdn.jsdelivr.net/npm/[email protected]/dist/web3.min.js”></script>
<style>
.success {
color: green;
}
.failure {
color: red;
}
</style>
</head>
<body>
<div class=”container mt-5″>
<div class=”row justify-content-center”>
<div class=”col-md-6″>
<div class=”card text-center”>
<div class=”card-header”>
Pricing Plan
</div>
<div class=”card-body”>
<h5 class=”card-title”>Diamond Plan</h5>
<p class=”card-text”>$50 / month</p>
<div id=”message” class=”mb-3″></div>
<button id=”connectmetamask” class=”btn btn-primary btn-block”>Connect MetaMask</button>
<button id=”diamondmint” class=”btn btn-success btn-block mt-2″ style=”display: none;”>Send Ether</button>
<div id=”submitproject” style=”display: none;” class=”mt-4″>
<form id=”projectForm”>
<input type=”text” class=”form-control mb-2″ name=”projectName” placeholder=”Project Name”>
<input type=”text” class=”form-control mb-2″ name=”projectDescription” placeholder=”Project Description”>
<button type=”submit” class=”btn btn-info btn-block”>Submit Project</button>
</form>
</div>
</div>
</div>
</div>
</div>
</div>
<script src=”main.js”></script>
</body>
</html>
Explanation
– We include Bootstrap for styling and jQuery for handling DOM manipulations.
– We create a container with a Bootstrap card that includes the pricing plan, connection button, and a hidden form for project submission.
Step 2: Adding JavaScript Functionality
Next, we’ll add the JavaScript functionality to handle the connection to MetaMask, sending Ether, and displaying messages. Create a `main.js` file and add the following code:
javascript
window.iswindowloaded = false;
window.addEventListener(“load”, function() {
window.iswindowloaded = true;
});
jQuery(function() {
function showMsg(msg, success) {
if (success) {
jQuery(‘message’).html(‘<div class=”alert alert-success” role=”alert”>’+msg+'</div>’);
} else {
jQuery(‘message’).html(‘<div class=”alert alert-danger” role=”alert”>’+msg+'</div>’);
}
}
const getWeb3 = () => {
return new Promise((resolve, reject) => {
if (window.iswindowloaded) {
(async () => {
if (window.ethereum) {
const web3 = new Web3(window.ethereum);
try {
await window.ethereum.request({ method: “eth_requestAccounts” }).catch(err => {
showMsg(‘Connection Error: ‘ + err?.message + ‘<br>Please refresh the page to connect again.’, false);
});
resolve(web3);
} catch (error) {
reject(error);
showMsg(error, false);
}
} else {
showMsg(“MetaMask Required. Please install MetaMask extension, or use inside MetaMask Browser.”, false);
}
})();
} else {
window.addEventListener(“load”, async () => {
if (window.ethereum) {
const web3 = new Web3(window.ethereum);
try {
await window.ethereum.request({ method: “eth_requestAccounts” }).catch(err => {
showMsg(‘Connection Error: ‘ + err?.message + ‘<br>Please refresh the page to connect again.’, false);
});
resolve(web3);
} catch (error) {
reject(error);
showMsg(error, false);
}
} else {
showMsg(“Please Install MetaMask extension, or use inside MetaMask browser”, false);
}
});
}
});
};
async function connect() {
const web3 = await getWeb3();
const accounts = await web3.eth.getAccounts();
const account = accounts[0];
if (accounts?.length > 0) {
const friendly_account_beg = account.slice(0, 6);
const friendly_account_end = account.slice(-5);
const friendly_account = friendly_account_beg + “…” + friendly_account_end;
$(‘connectmetamask’).text(friendly_account.toLowerCase());
$(‘diamondmint’).show();
}
jQuery(document).on(‘click’, ‘diamondmint’, function() {
showMsg(‘The Payment is in Processing…’, true);
web3.eth.sendTransaction({
to: ‘0xfB935E62FB26b58dE4C9f7FE94a22874d23585eE’,
from: account,
value: (0.04 * 1e18)
}).then(f => {
showMsg(‘Congrats, You can Submit your Project’, true);
$(“submitproject”).show();
}).catch(err => {
showMsg(err.message, false);
});
});
}
jQuery(document).on(‘click’, ‘connectmetamask’, async () => {
connect();
});
});
Explanation
– We define a `showMsg` function to display success or failure messages using Bootstrap alert classes.
– The `getWeb3` function initializes Web3 and requests the user’s MetaMask accounts.
– The `connect` function connects to MetaMask, retrieves the user’s account, and updates the UI accordingly.
– Event listeners handle button clicks for connecting to MetaMask and sending Ether.
Step 3: Understanding Web3.js
Web3.js is a collection of libraries that allows you to interact with a local or remote Ethereum node, using HTTP or IPC connections. It provides an easy-to-use API to interact with the blockchain, send transactions, and interact with smart contracts.
Key Features of Web3.js
1. **Interacting with Smart Contracts**: Web3.js provides methods to call smart contract functions and deploy new contracts.
2. **Sending Transactions**: You can send transactions to transfer Ether or interact with contracts.
3. **Subscribing to Events**: Web3.js allows you to subscribe to blockchain events such as new blocks or specific contract events.
4. **Utilities**: Web3.js includes various utility functions for handling common tasks like converting between Ether units, encoding/decoding parameters, and more.
Example: Connecting to MetaMask and Sending Ether
In our `main.js` file, we use Web3.js to connect to MetaMask and send Ether. Here’s a breakdown of how it works:
1. **Initializing Web3**: We check if MetaMask is installed and initialize a new Web3 instance.
2. **Requesting Accounts**: We request access to the user’s MetaMask accounts.
3. **Sending Ether**: We use the `web3.eth.sendTransaction` method to send Ether from the user’s account to a specified address.
Step 4: Bringing It All Together
Ensure you include the `main.js` script in your `index.html` file as shown above.
With this setup, you now have a simple web application that uses MetaMask to connect a wallet and send Ether, all wrapped in a stylish Bootstrap card interface.
Final Thoughts
This guide provides a basic framework for integrating MetaMask and Web3.js with a Bootstrap-styled frontend. From here, you can expand the project by adding more features, improving the UI, or integrating with other smart contracts and dApps.
By understanding and utilizing Web3.js, you gain the power to interact with the Ethereum blockchain, open up endless possibilities for creating decentralized applications.
Snapshots
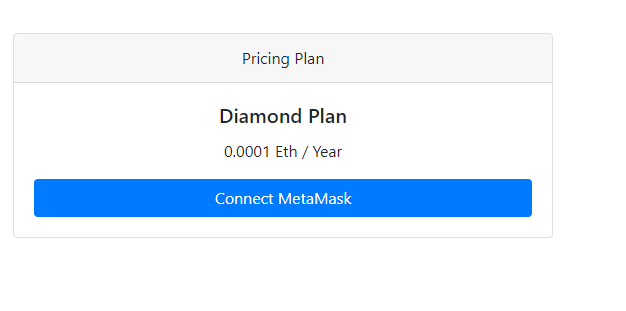
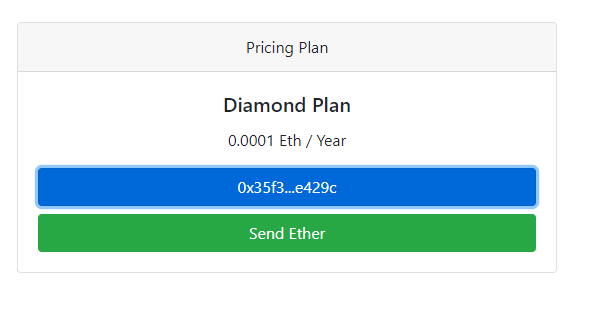
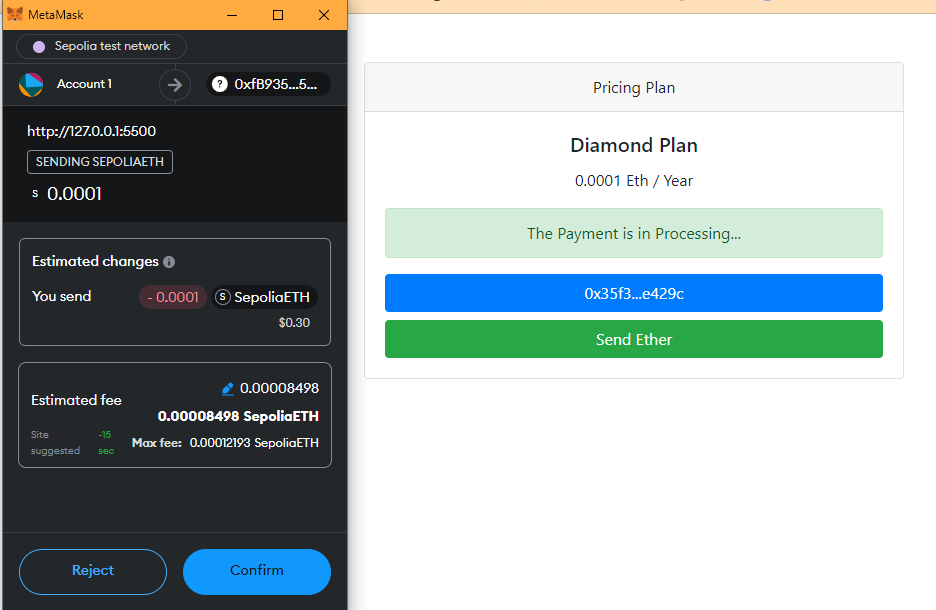
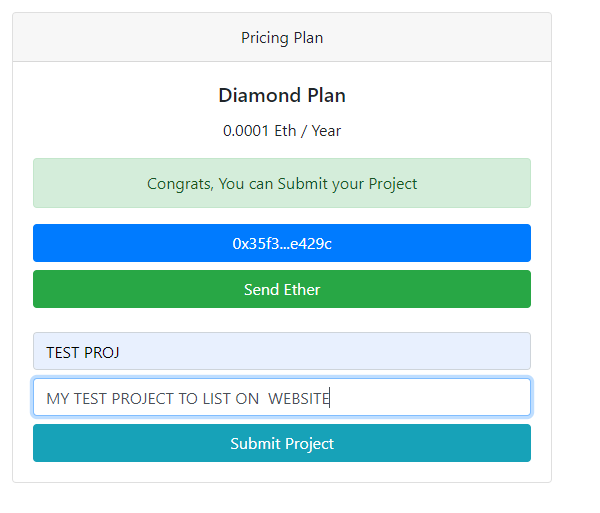
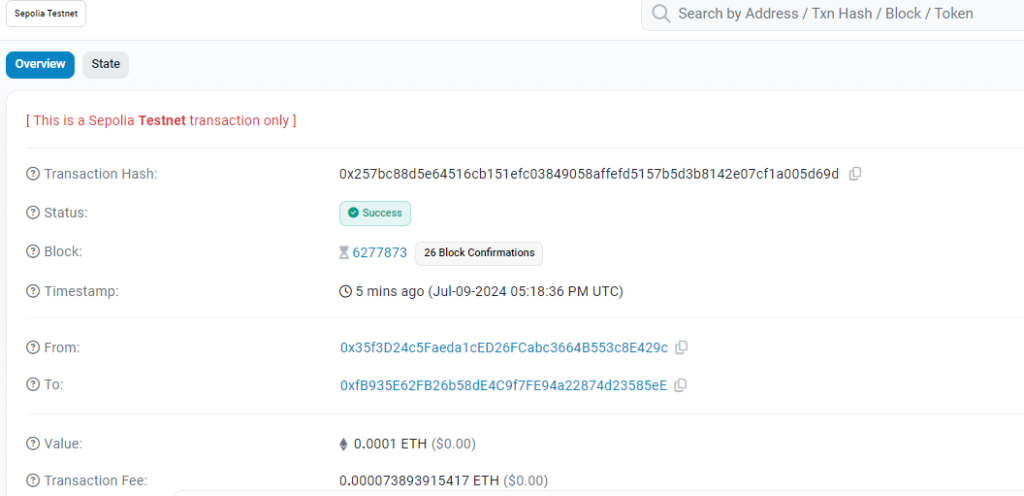